Excel HubSpot integration: An introduction for VBA programmers and business users
If you have some VBA experience, are an Excel superuser or a business leader looking to integrate Excel with HubSpot, this guide is designed with you in mind. The ability to connect different software platforms effectively is increasingly important in our data-centric world. This guide will provide a straightforward introduction to using VBA for Excel HubSpot integration – without any costly third-party tools.
In this post, we'll begin by showing how to send data from Excel to HubSpot. We'll demonstrate this by creating a new deal in HubSpot directly from Excel. Following this, we'll illustrate how to retrieve data from HubSpot into Excel using VBA. This way, you'll gain an understanding of how to synchronize your data between the two platforms, facilitating efficient data management and thus opening up for building powerful Excel solutions using your HubSpot CRM data.
By leveraging the HubSpot Excel API and HubSpots private apps, we can create a useful connection between these two powerful tools, opening up new opportunities for data analysis, customer relationship management, and business intelligence. Let's explore the practicalities and potential of Excel VBA and HubSpot integration together.
Benefits of using VBA to integrate Excel with HubSpot
Business leaders know the value of leveraging existing resources to streamline processes and gain a competitive edge. And one of the most underutilized resources in many businesses is Excel, a tool already installed on close to every office computer. When combined with Visual Basic for Applications (VBA), Excel becomes a powerful platform for integrating with HubSpot. Here's why this integration could be a game-changer for your business:
- Affordability: With VBA, you can create a custom integration solution that's more cost-effective in the long run. Once the initial development is done, there are no ongoing third-party application fees to worry about.
- Leveraging Excel's capabilities: Excel is one of the most widely used data analysis tools in the world. It's robust, flexible, and powerful. By integrating HubSpot with Excel, you can use familiar Excel functions and features to analyze your HubSpot data.
- Automation: VBA allows you to automate tasks, saving time and reducing the potential for manual errors. This can be particularly useful for repetitive tasks like updating data or generating reports.
- Customization: Every business is unique, and so are its data needs. With VBA, you can build a custom solution that fits your specific requirements, rather than trying to adjust your processes to fit a third-party tool.
- Improved Data Management: HubSpot is an excellent CRM tool, but it becomes even more powerful when you can effectively connect it with other data in your organization. By integrating with Excel, you can more easily combine and compare HubSpot data with other business data to gain new insights.
- Better Reporting: By bringing HubSpot data into Excel, you can create more complex and tailored reports than you might be able to within HubSpot alone. Excel's broad range of data visualization tools can help bring your HubSpot data to life.
- Increased Productivity: By reducing manual data entry and improving data accessibility, integrating Excel and HubSpot can help increase your team's productivity.
- Scalability: A VBA solution can be expanded and modified as your business grows and changes, making it a scalable solution that can adapt to your evolving needs.
Setting the Stage: Preliminary Requirements for Excel-HubSpot Integration
Before we start with any VBA code, we need to set up a few things in HubSpot. This is much simpler than it sounds, and we'll guide you through each step.
First, we need to create a Private App in your HubSpot account. A private app lets you use HubSpot's APIs to communicate with your specific HubSpot data. Here's how you can do it:
Log into your HubSpot account and click on the settings icon in the main navigation bar.
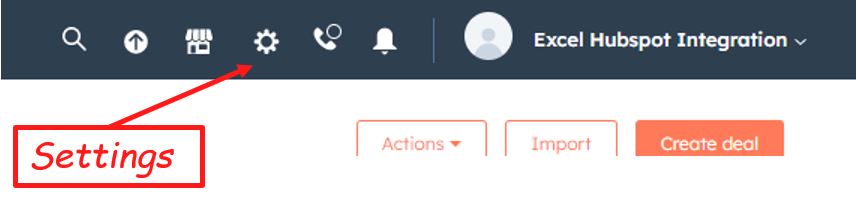
Navigate to "Integrations ⊳ Private Apps" in the left sidebar menu.
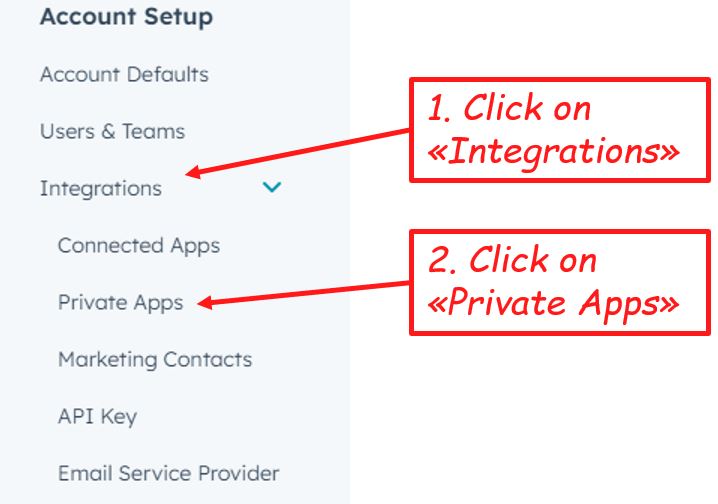
This will bring you to the private app page. Here, you can see and manage your private HubSpot apps or create a new one. Click on "Create a private app" to continue.
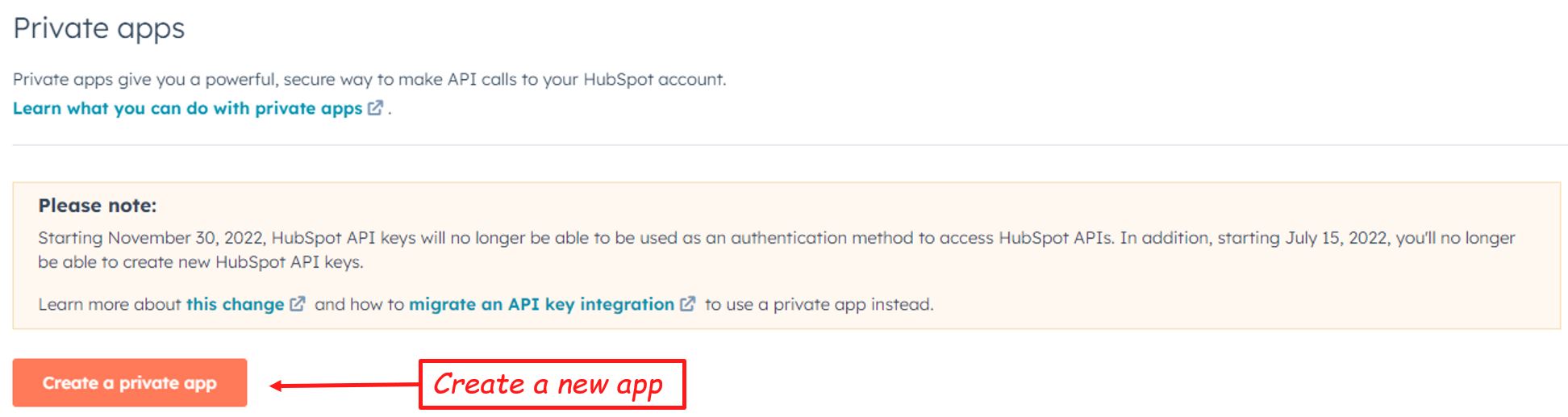
Now, you'll be on the "Basic Info" tab. Here, you'll need to enter your app's name, upload a logo, and provide a brief description of your app. This will help you recognize it later.
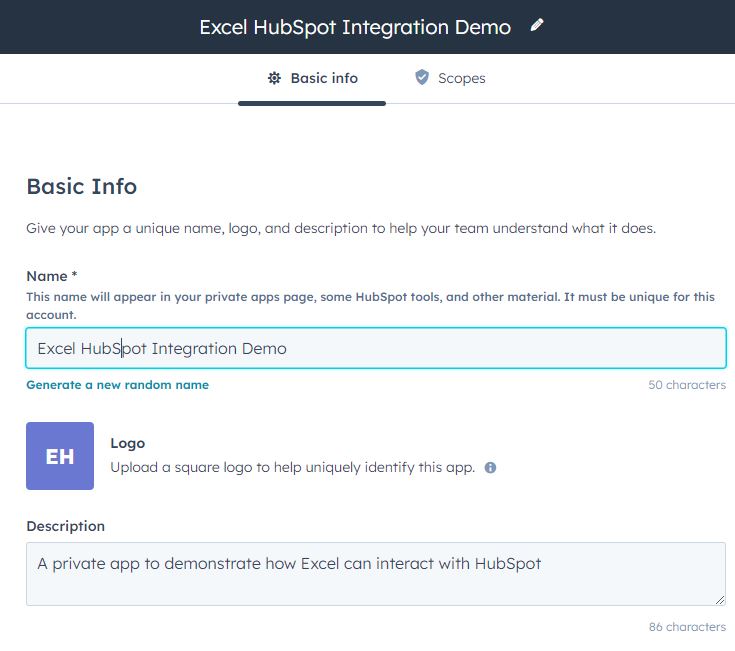
Go to the "Scopes" tab. Here, you'll need to select the Read or Write checkboxes for each scope you want your private app to access. In simpler terms, this is where you decide what data your app can read or modify. You can also use the "Find a scope" search bar if you're looking for a specific scope.
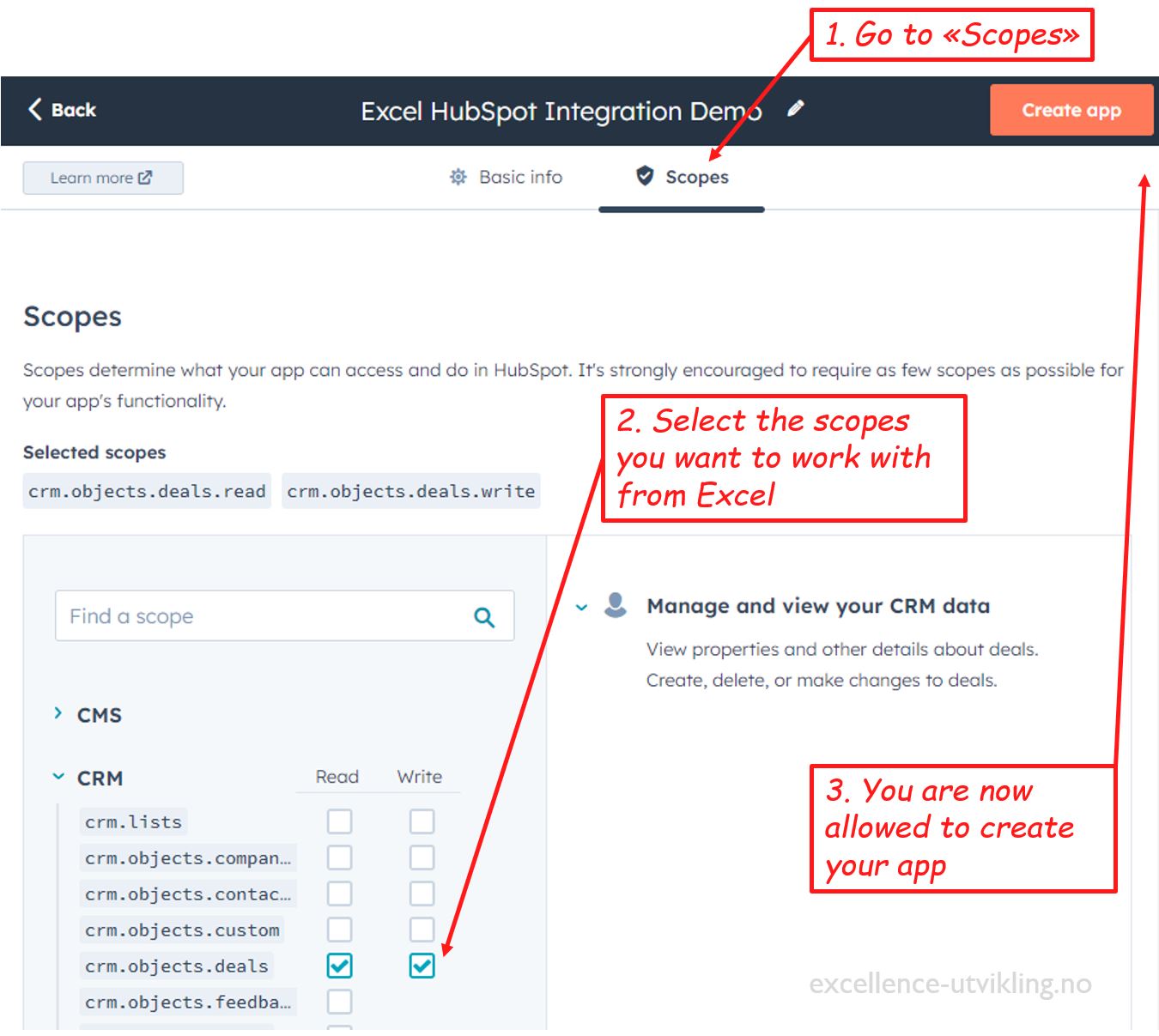
Once you've configured your app to your liking, click on "Create app" in the top right. Confirm the popup that will come up afterwards.
For more detailed information, you can refer to the HubSpot's documentation on creating a private app.
Second, we need to obtain your app's Access Token. This is essentially your app's password when it communicates with HubSpot's servers, so make sure to keep it safe!
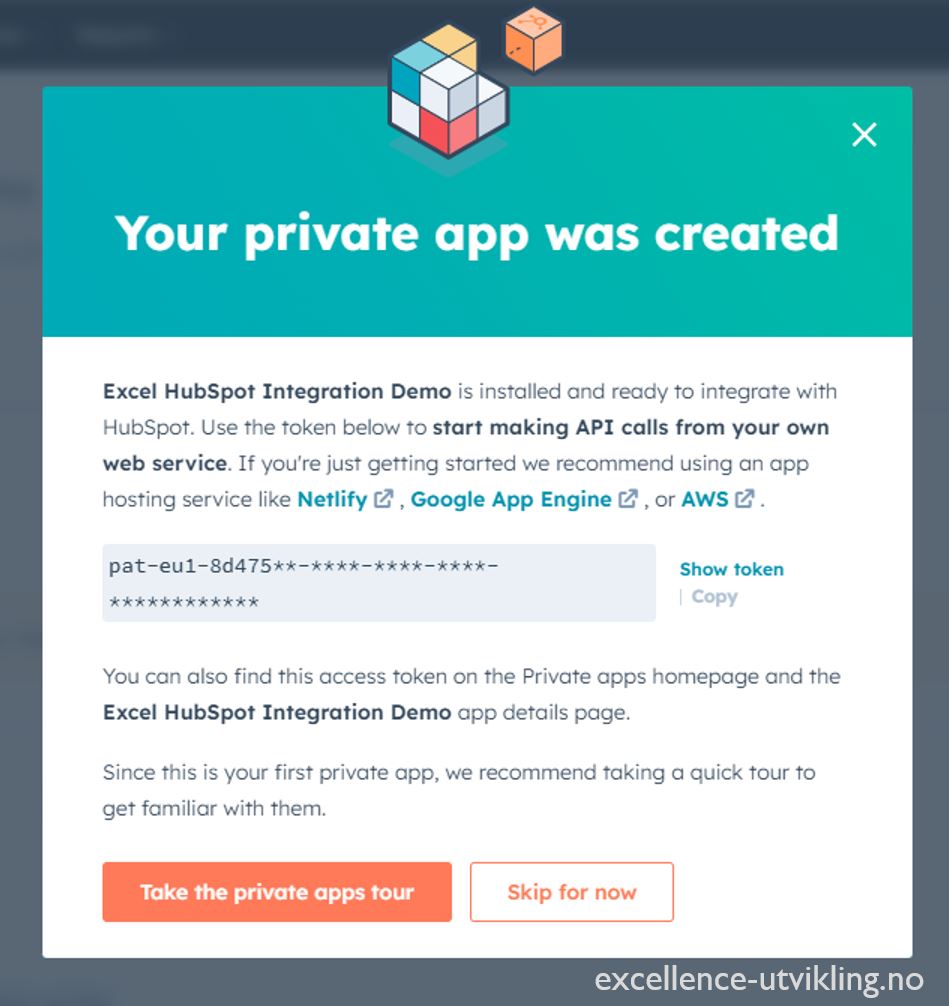
Remember, treat your access tokens as you would any sensitive information. Never expose them publicly, as they give access to your HubSpot data. If you suspect someone might haven gotten access to your token, you can receive a new token on your apps page.
Now that we've got the preliminary requirements covered, we can dive into the exciting part: coding the integration!
Sending Data from Excel to HubSpot using VBA and the HubSpot API
Great, we're all set to make our first API call! In this section, we'll explore how to send data from Excel to HubSpot using VBA, with the example of creating a (very basic) deal. In essence, we will use an HTTP POST request, which is a way of sending data to a server. In this case, our server is HubSpot's CRM.
Here's a simple VBA code that does exactly that:
Sub CreateNewDeal() ' ---------------------------------------------------------------- ' Purpose: This macro communicates with the HubSpot CRM API using the HTTP POST method and creates a very simple deal ' Author: Michael Markus Wycisk / Excellence Utvikling AS ' Created Date: 23.05.2023 ' Change log: ' ---------------------------------------------------------------- Dim objHttp As Object Dim sSecretToken As String Dim sUrl As String Dim sBody As String ' Create a new instance of the MSXML2.ServerXMLHTTP object. ' We uses late-binding, so you should not need to add any new references to this project Set objHttp = CreateObject("MSXML2.ServerXMLHTTP") ' Set your secret access token and the HubSpot API URL sSecretToken = "pat-eu1-8d475**-****-****-****-************" sUrl = "https://api.hubapi.com/crm/v3/objects/deals" ' Define the deal properties to be sent in the json request body ' We create a very simple deal just to show the concept sBody = "{""properties"": {""dealname"": ""Example customer 1"", ""amount"": ""4500"", ""dealstage"": ""closedwon""}}" ' Open a connection to the HubSpot API objHttp.Open "POST", sUrl, False ' Set the request headers. objHttp.setRequestHeader "Authorization", "Bearer " & sSecretToken objHttp.setRequestHeader "Content-Type", "application/json" ' Send the POST request objHttp.send (sBody) ' Display the status and response text Debug.Print "Status: " & objHttp.Status Debug.Print "Response: " & objHttp.responseText End Sub
This code does a few things. It first sets up a new HTTP request using the MSXML2.ServerXMLHTTP
object. It then sets your secret access token and the URL of the HubSpot API endpoint you want to communicate with.
The sBody
variable contains the data that will be sent to HubSpot to create the deal. This is a JSON string that specifies the deal's name, amount, and stage. Of course in a "real world" example, having these values hard coded would often make little to no sense. But of course, you can imagine that you can read these variables from a UserForm, cells in your Excel sheet, some external sources - you name it! But the scope of this post if to give a basic introduction to using HubSpots API, so we keep things as easy as possible.
Next, the code opens a connection to the API and sets the necessary headers, including your access token. This is where that token you obtained earlier comes into play.
After everything is set up, the code sends the POST request. The response from the server (i.e., HubSpot) is then printed to the debug window in VBA, showing the status of the request and any response text.
I real use case, you should of course also add solid error handling, as things could go wrong:
- misconfigurations in the private app
- errors in internet connection
- expired or invalid access token
- incorrect data format or values
- server-side issues
- rate limit exceeded
You can use the return values of the request to check for success. If the deals where created, you will see that objHttp.Status = 201
. In a solid error handling, you might check your respons code to some of the response codes that HubSpot list here.
Retrieving deal data from HubSpot using Excel and VBA
In this section, we will now look into the process of retrieving data from the HubSpot CRM API. We will focus on retrieving the deal data that we previously created. But of course, you can absolutely use this process to read all your other deals as well and use them in your Excel reports.
Sub GetHubSpotData() ' ---------------------------------------------------------------- ' Purpose: This macro retrieves data from the HubSpot CRM API using the HTTP GET method ' Author: Michael Markus Wycisk / Excellence Utvikling AS ' Created Date: 19.05.2023 ' Change log: ' ---------------------------------------------------------------- Dim objHttp As Object Set objHttp = CreateObject("MSXML2.ServerXMLHttp") Dim sUrl As String Dim sSecretToken As String ' Secret Hubspot token - Replace this token with you secret token sSecretToken = "pat-eu1-8d475**-****-****-****-************" ' API endpoint to get deals data sUrl = "https://api.hubapi.com/crm/v3/objects/deals" objHttp.Open "GET", sUrl, False ' Set the request headers. objHttp.setRequestHeader "Authorization", "Bearer " & sSecretToken objHttp.setRequestHeader "Content-Type", "application/json" ' Send the request and wait for the response. objHttp.send ' Output the response to the Immediate Window Debug.Print objHttp.responseText End Sub
The process of retrieving data from the HubSpot CRM API involves making an HTTP GET request to the appropriate API endpoint. In our case, we are interested in the deals data, so we will make our request to https://api.hubapi.com/crm/v3/objects/deals
. This is done using the Open method of the MSXML2.ServerXMLHttp
object.
We then set the necessary request headers using the setRequestHeader
method, including our authorization token and the content type.
After sending the request using the send
method, the responseText
property of the objHttp
object will contain the JSON response from the HubSpot API. We output this response to the Immediate Window as proof of concert.
The JSON response from HubSpot will contain an array of deal objects under the results
key. Each deal object will have its unique id
and a properties
object containing details about the deal such as the amount
, dealname
, dealstage
, and pipeline
. It also includes timestamps for when the deal was createdAt
and updatedAt
, and a flag archived
indicating whether the deal is archived.
{ "results": [ { "id": "7536754135", "properties": { "amount": "4500", "closedate": null, "createdate": "2023-05-23T17:33:45.535Z", "dealname": "Example customer 1", "dealstage": "closedwon", "hs_lastmodifieddate": "2023-05-23T17:33:46.276Z", "hs_object_id": "7536754135", "pipeline": "default" }, "createdAt": "2023-05-23T17:33:45.535Z", "updatedAt": "2023-05-23T17:33:46.276Z", "archived": false } ] }
To parse this JSON data in VBA, you can use tools like the JsonConverter on GitHub.
How to treat JSON data in VBA would be a blog post in itself and go beyond the scope of this article. However, there is a variety of good blog post out on the web. Here you can find a simple guide on using the JsonConverter.
Navigating potential pitfalls and understanding API limitations
While interacting with HubSpot's API, it's crucial to be aware of potential issues that might arise. These can range from rate limits enforced by HubSpot, inconsistencies in data formatting, to unforeseen errors in the response from the API.
HubSpot, like many other service providers, imposes rate limits on their APIs to ensure fair usage and maintain the stability of their services. Exceeding these limits might result in your requests being throttled or denied, disrupting your application's functionality.
Data formatting is another aspect that can lead to complications. For instance, if the data you're sending doesn't match the expected format by HubSpot's API, you might encounter errors. Conversely, when receiving data, it's important to correctly parse and handle the response to avoid issues.
Unforeseen errors can always occur when working with APIs. These could stem from a variety of sources, such as network issues, temporary service unavailability, or changes in the API endpoints or data schemas.
Given these potential pitfalls, it's recommended to implement robust error handling in your code, as we've touched on earlier. Additionally, regularly consulting the HubSpot API documentation and keeping an eye on the error messages returned by the API will help you troubleshoot issues and ensure that your application runs smoothly. By understanding and anticipating these challenges, you can create more resilient and reliable applications that interact with the HubSpot API.
Conclusion - Excel/VBA and HubSpot - A powerful combination
In this article, we've taken a deep dive into how Excel/VBA can be combined with HubSpot's powerful APIs to create robust, efficient, and practical business solutions. We've looked at how to set up a private app in HubSpot, authenticate with an access token, and make API calls to create, read, update, and delete deal records in the HubSpot CRM.
The benefits of such an integration are manifold. By automating data transfers between Excel and HubSpot, you can save valuable time, reduce the potential for human error, and make your processes more efficient. For businesses that heavily rely on both Excel for data analysis and HubSpot for customer relationship management, this integration can be a game-changer, streamlining workflows and improving productivity.
As always, it's important to be aware of potential pitfalls and limitations when working with APIs, including rate limits and data formatting issues. But with careful planning, diligent error handling, and a solid understanding of the API documentation, these challenges can be effectively managed.
In conclusion, the integration of Excel/VBA with HubSpot's API opens up a world of possibilities for enhancing your business processeses. This integration demonstrates how even established technologies like Excel/VBA can be combined with modern platforms like HubSpot to create functional and efficient workflows.
Partnering for Success: Your Excel Consultant
At Excellence Utvikling AS, we understand the power of Excel and the transformative potential it holds for your business. With extensive experience as an Excel consultant, we specialize in integrating Excel/VBA solutions with platforms like HubSpot, allowing you to streamline your operations and enhance data management.
Our Excel consulting services extend beyond just integrations. We offer tailored solutions for automating your Excel sheets, writing custom VBA macros, and developing intricate Excel models. Whether it's designing business templates or tackling complex data challenges, our goal is to enable you to make the most of your data.
If you're ready to unlock the full potential of Excel in your business, don't hesitate to reach out. We're here to guide you through every step of the process, and we're excited to partner with you to achieve your business goals.
Disclaimer
Please be aware that while we strive to provide accurate and useful information, we cannot guarantee the accuracy, reliability, or completeness of the information presented in this blog post. The content provided here is for informational purposes only. We have provided external links and code examples for your convenience, but we are not responsible for the content, accuracy, or operation of any external sites or the results of using the provided code. Users are advised to exercise due diligence and caution while using the provided code and visiting external sites. Also, while we make all reasonable efforts to ensure that the code provided in our examples is correct, we cannot foresee all possible scenarios and use cases. Therefore, errors may occur, and users should carefully test any code before implementing it in a production environment. By using any information from this blog post, you agree to do so at your own risk, and you accept full responsibility for any consequences resulting from such use.